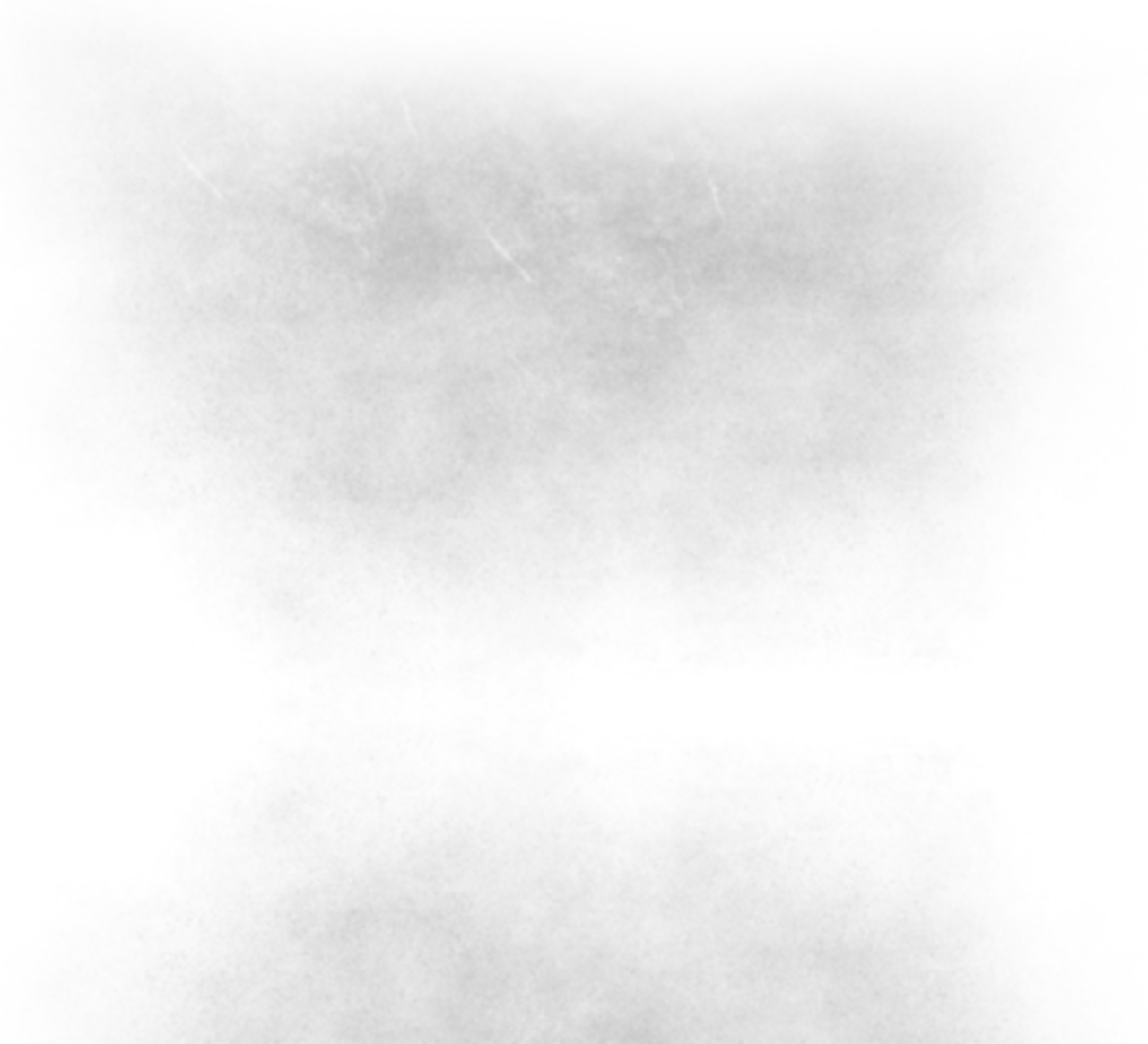

C#
Codigo C#:
Capas:
EXAMEN.Datos
EXAMEN.Entidad
EXAMEN.Negocio
EXAMEN.Presentacion
EXAMEN.Datos:
AlumnosDL.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using EXAMEN.Entidad;
using Examen.Data;
namespace EXAMEN.Datos
{
public class AlumnosDL
{
public DataSet ListarAlumnos()
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_ListarAlumnos", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
SqlDataAdapter adapter = new SqlDataAdapter(cmd);
conexion.AbrirConexionBD();
DataSet dsVehiculos = new DataSet();
adapter.Fill(dsVehiculos, "Vehiculos");
return dsVehiculos;
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
public void InsertarAlumnos(AlumnosBE alumnos)
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_InsertarAlumnos", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add(new SqlParameter("@nombre", alumnos.Nombre));
cmd.Parameters.Add(new SqlParameter("@telefono", alumnos.Telefono));
cmd.Parameters.Add(new SqlParameter("@nota1", alumnos.Nota1));
cmd.Parameters.Add(new SqlParameter("@nota2", alumnos.Nota2));
cmd.Parameters.Add(new SqlParameter("@nota_final", alumnos.Nota_final));
cmd.Parameters.Add(new SqlParameter("@gama", alumnos.Gama.IdGama));
conexion.AbrirConexionBD();
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
public void ActualizarAlumnos(AlumnosBE alumnos)
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_ActualizarAlumnos", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add(new SqlParameter("@nombre", alumnos.Nombre));
cmd.Parameters.Add(new SqlParameter("@telefono", alumnos.Telefono));
cmd.Parameters.Add(new SqlParameter("@nota1", alumnos.Nota1));
cmd.Parameters.Add(new SqlParameter("@nota2", alumnos.Nota2));
cmd.Parameters.Add(new SqlParameter("@nota_final", alumnos.Nota_final));
cmd.Parameters.Add(new SqlParameter("@gama", alumnos.Gama.IdGama));
conexion.AbrirConexionBD();
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
public void EliminarAlumnos(string nombre)
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_DeleteAlumnos", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add(new SqlParameter("@nombre", nombre));
conexion.AbrirConexionBD();
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
public AlumnosBE ListarAlumnosPorNombre(string Nombre)
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_ListarAlumnosPorNombre", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
cmd.Parameters.Add(new SqlParameter("@nombre", Nombre));
conexion.AbrirConexionBD();
SqlDataReader reader = cmd.ExecuteReader();
AlumnosBE alumnos = new AlumnosBE(); ;
if (reader.Read())
{
alumnos.Nombre = Convert.ToString(reader["nombre"]);
alumnos.Telefono = Convert.ToString(reader["telefono"]);
alumnos.Nota1 = Convert.ToString(reader["nota1"]);
alumnos.Nota2 = Convert.ToString(reader["nota2"]);
alumnos.Nota_final = Convert.ToString(reader["nota_final"]);
alumnos.Gama.IdGama = Convert.ToInt32(reader["gama"]);
}
return alumnos;
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
}
}
ConexionDL.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using System.Data.Common;
using System.Configuration;
namespace Examen.Data
{
public class ConexionDL
{
private SqlConnection con; // Obj Conexion
public SqlConnection Con
{
get { return con; }
set { con = value; }
}
public ConexionDL()
{
/* Para trabajar con el servidor SQL de la maquina */
string miCadenaConexion = ConfigurationManager.ConnectionStrings["ExamenBD"].ConnectionString; // PARA QUE ESTO FUNCIONE TENGO QUE AGREGAR LA REFERENCIA DE SYSTEM.CONFIGURATION DE .NET
Con = new SqlConnection(miCadenaConexion);
}
/// <summary>
/// Metodo para Abrir la Conexion
/// </summary>
public void AbrirConexionBD()
{
Con.Open();
}
/// <summary>
/// Metodo para Cerrar la Conexion
/// </summary>
public void CerrarConexionBD()
{
Con.Close();
}
public DataSet ListarAlumnos()
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_ListarVehiculos", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
SqlDataAdapter adapter = new SqlDataAdapter(cmd);
conexion.AbrirConexionBD();
DataSet dsVehiculos = new DataSet();
adapter.Fill(dsVehiculos, "Vehiculos");
return dsVehiculos;
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
}
}
GamaDL.cs:
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using EXAMEN.Entidad;
namespace Examen.Data
{
public class GamaDL
{
public List<GamaBE> ListarGamas()
{
ConexionDL conexion = new ConexionDL();
try
{
SqlCommand cmd = new SqlCommand("sp_ListarGamas", conexion.Con);
cmd.CommandType = CommandType.StoredProcedure;
conexion.AbrirConexionBD();
SqlDataReader reader = cmd.ExecuteReader();
List<GamaBE> listaGamas = new List<GamaBE>();
while (reader.Read())
{
GamaBE gama = new GamaBE();
gama.IdGama = Convert.ToInt32(reader["IdGama"]);
gama.DescripcionGama = Convert.ToString(reader["Descripcion"]);
gama.Precio = Convert.ToDecimal(reader["Precio"]);
listaGamas.Add(gama);
}
return listaGamas;
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
finally
{
conexion.CerrarConexionBD();
}
}
}
}
EXAMEN.Entidad:
AlumnosBE.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace EXAMEN.Entidad
{
public class AlumnosBE
{
string nombre;
public string Nombre
{
get { return nombre; }
set { nombre = value; }
}
string telefono;
public string Telefono
{
get { return telefono; }
set { telefono = value; }
}
string nota1;
public string Nota1
{
get { return nota1; }
set { nota1 = value; }
}
string nota2;
public string Nota2
{
get { return nota2; }
set { nota2 = value; }
}
string nota_final;
public string Nota_final
{
get { return nota_final; }
set { nota_final = value; }
}
GamaBE gama;
public GamaBE Gama
{
get { return gama; }
set { gama = value; }
}
public AlumnosBE()
{
gama = new GamaBE();
}
public AlumnosBE(string _nombre, string _telefono, string _nota1, string _nota2, string _nota_final,
GamaBE _gama)
{
nombre = _nombre;
telefono = _telefono;
nota1 = _nota1;
nota2 = _nota2;
nota_final = _nota_final;
gama = _gama;
}
}
}
GamaBE.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace EXAMEN.Entidad
{
public class GamaBE
{
int idGama;
public int IdGama
{
get { return idGama; }
set { idGama = value; }
}
string descripcionGama;
public string DescripcionGama
{
get { return descripcionGama; }
set { descripcionGama = value; }
}
decimal precio;
public decimal Precio
{
get { return precio; }
set { precio = value; }
}
public GamaBE()
{
}
public GamaBE(int _idGama, string _descripcionGama, decimal _precio)
{
idGama = _idGama;
descripcionGama = _descripcionGama;
precio = _precio;
}
}
}
EXAMEN.Negocio:
AlumnosBL.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using Examen.Data;
using EXAMEN.Entidad;
using EXAMEN.Datos;
namespace EXAMEN.Negocio
{
public class AlumnoBL
{
public DataSet ListarAlumnos()
{
AlumnosDL alumnosDL = new AlumnosDL();
return alumnosDL.ListarAlumnos();
}
public void InsertarAlumnos(AlumnosBE alumnos)
{
AlumnosDL alumnosDL = new AlumnosDL();
alumnosDL.InsertarAlumnos(alumnos);
}
public void ActualizarAlumnos(AlumnosBE alumnos)
{
AlumnosDL alumnosDL = new AlumnosDL();
alumnosDL.ActualizarAlumnos(alumnos);
}
public void EliminarVehiculo(string nombre)
{
AlumnosDL alumnosDL = new AlumnosDL();
alumnosDL.EliminarAlumnos(nombre);
}
public void ListarAlumnosporNombre(string nombre)
{
AlumnosDL alumnosDL = new AlumnosDL();
alumnosDL.ListarAlumnosPorNombre(nombre);
}
}
}
GamaBL.cs:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using Examen.Data;
using EXAMEN.Entidad;
namespace Examen.Negocio
{
public class GamaBL
{
public List<GamaBE> ListarGamas()
{
GamaDL gamaDL = new GamaDL();
return gamaDL.ListarGamas();
}
}
}
EXAMEN.Presentacion:
App.config:
ESTE FUNCIONA EN LA CASA:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
</configSections>
<connectionStrings>
<add name="ExamenBD" connectionString="Data Source=Moty; Initial Catalog=EXAMEN; Integrated Security = True"
providerName="System.Data.SqlClient" />
</connectionStrings>
<!--<add name = "CaboBD"
connectionString = "Data source= 10.20.0.3; Initial Catalog = CABO; UserID = sa; Password = '123456'"
providerName ="System.data.SqlClient" />-->
</configuration>
<!--ESTE FUNCIONA EN EL LABORATORIO-->
<!--<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
</configSections>
<connectionStrings>
<add name="CaboBD"
connectionString="Data Source=localhost;Initial Catalog=CABO;User ID=sa;Password=123456"
providerName="System.Data.SqlClient" />
</connectionStrings>
--><!--<add name = "CaboBD"
connectionString = "Data source= 10.20.0.3; Initial Catalog = CABO; UserID = sa; Password = '123456'"
providerName ="System.data.SqlClient" />--><!--
</configuration>-->
ESTE OTRO TAMBIEN:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
</configSections>
<connectionStrings>
<add name="CaboBD"
connectionString="Data Source=localhost;Initial Catalog=CABO;User ID=sa;Password=123456"
providerName="System.Data.SqlClient" />
</connectionStrings>
<!--<add name = "CaboBD"
connectionString = "Data source= 10.20.0.3; Initial Catalog = CABO; UserID = sa; Password = '123456'"
providerName ="System.data.SqlClient" />-->
</configuration>
ESTO VA EN EL CODIGO DEL FORM :
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Imaging;
using System.IO;
using EXAMEN.Entidad;
using Examen.Negocio;
using EXAMEN.Negocio;
namespace EXAMEN.Presentacion
{
public partial class Form3 : Form
{
public Form3()
{
InitializeComponent();
RefrescarGrid();
CargarGamas();
}
void CargarGamas()
{
GamaBL gamaBL = new GamaBL();
List<GamaBE> lista = gamaBL.ListarGamas();
//Vaciar comboBox
cmb_gama.DataSource = null;
//Asignar la propiedad DataSource
cmb_gama.DataSource = lista;
//Indicar qué propiedad se verá en la lista
cmb_gama.DisplayMember = "DescripcionGama";
//Indicar qué valor tendrá cada Ãtem
cmb_gama.ValueMember = "IdGama";
}
void RefrescarGrid()
{
AlumnoBL vehiculoBL = new AlumnoBL();
dataGridView1.DataSource = vehiculoBL.ListarAlumnos().Tables["Vehiculos"];
//dataGridView1.Columns["nombre"].Visible = false;
//dataGridView1.Columns["gama"].Visible = false;
//dataGridView1.Columns["telefono"].Visible = false;
//dataGridView1.Columns["nota1"].Visible = false;
dataGridView1.Columns["nombre"].HeaderText = "Nombre";
dataGridView1.Columns["telefono"].HeaderText = "Telefono";
dataGridView1.Columns["nota1"].HeaderText = "Nota1";
dataGridView1.Columns["nota2"].HeaderText = "Nota2";
dataGridView1.Columns["nota_final"].HeaderText = "Nota_final";
}
private void tsb_nuevo_Click(object sender, EventArgs e)
{
txt_nombre.Enabled = true;
tsb_guardar.Enabled = true;
}
private void tsb_guardar_Click(object sender, EventArgs e)
{
try
{
AlumnosBE vehiculo = new AlumnosBE(txt_nombre.Text, txt_telefono.Text, txt_nota1.Text, txt_nota2.Text, txt_notafinal.Text,
(GamaBE)cmb_gama.SelectedItem);
AlumnoBL vehiculoBL = new AlumnoBL();
vehiculoBL.InsertarAlumnos(vehiculo);
MessageBox.Show("Vehiculo Insertado Exitosamente");
RefrescarGrid();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
private void dataGridView1_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
{
//LimpiarControles();
string nombre = dataGridView1.Rows[e.RowIndex].Cells["nombre"].Value.ToString();
AlumnoBL vehiculoBL = new AlumnoBL();
AlumnosBE vehiculoBE = new AlumnosBE();
txt_nombre.Text = vehiculoBE.Nombre;
txt_telefono.Text = vehiculoBE.Telefono;
txt_nota1.Text = vehiculoBE.Nota1;
txt_nota2.Text = vehiculoBE.Nota2;
txt_notafinal.Text = vehiculoBE.Nota_final;
}
private void tsb_crear_Click(object sender, EventArgs e)
{
try
{
RefrescarGrid();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
private void tsb_eliminar_Click(object sender, EventArgs e)
{
AlumnoBL alumnoBL = new AlumnoBL();
alumnoBL.EliminarVehiculo(txt_nombre.Text); // LO BORRA DE ACUERO AL NOMBRE DEL TEXTBOX
MessageBox.Show("Vehiculo Eliminado Exitosamente");
RefrescarGrid();
}
}
}