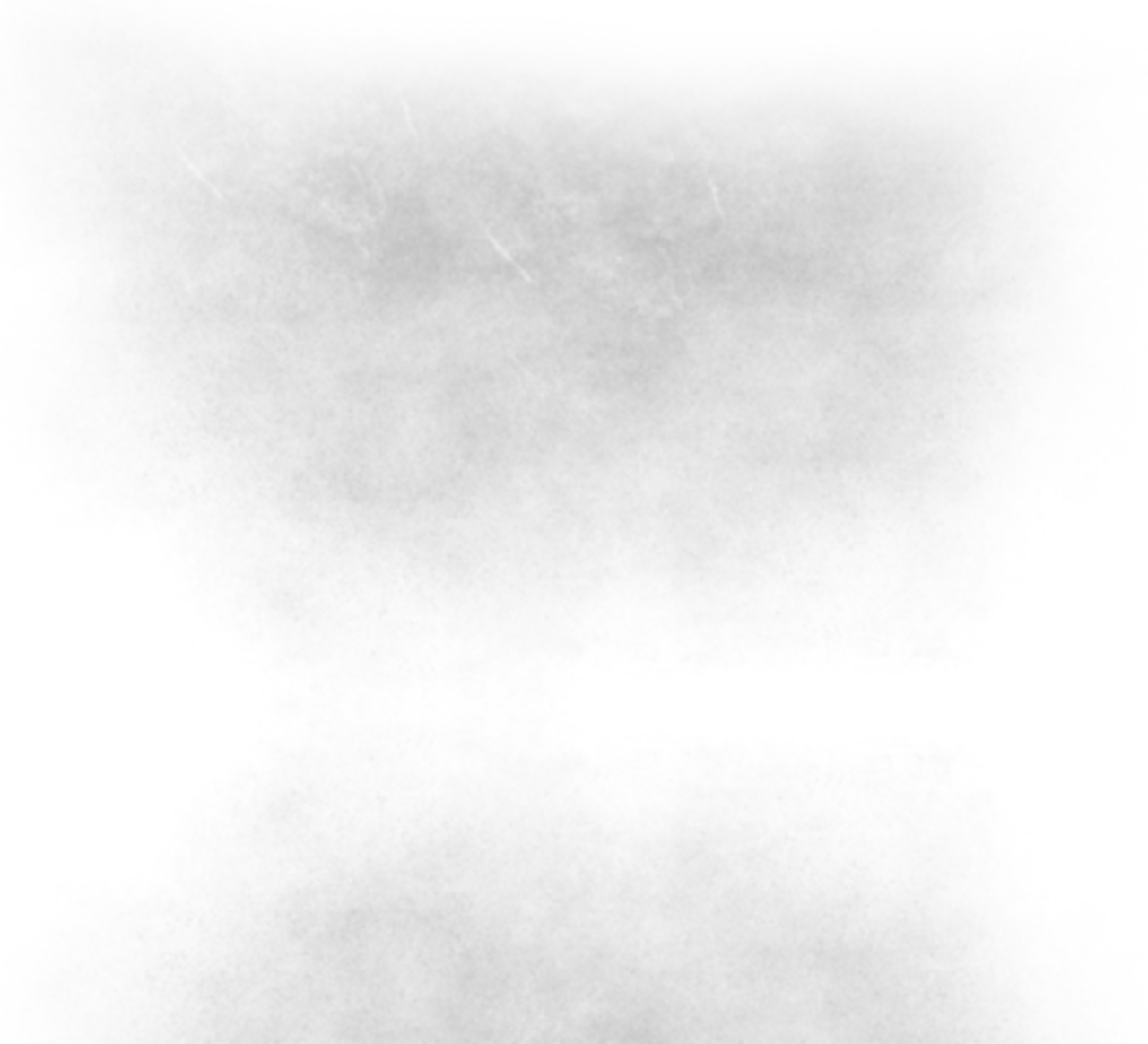
1 - JAVA
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string palabra;
palabra = textBox1.Text;
// string[] palabras = palabra.Split(new char[] { ' ' });
string[] palabras = palabra.Split();
Array.Reverse(palabras);
string reverseTxt = "";
for (int i = 0; i <= palabras.Length - 1; i++)
{
//reverseTxt += palabras.GetValue(i) + " ";
reverseTxt += palabras[i] + " ";
}
this.textBox2.Text = reverseTxt;
}
}
}
2 - PHYTON
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string palabra = textBox1.Text;
string reverseTxt = " ";
// string[] palabras = palabra.Split(new char[]{ ' ' });
char[] palabras = palabra.ToCharArray();
for (int i = 0; i < palabras.Length; i++)
{
if (palabras[i] == 'a')
{
reverseTxt += '2';
}
else if (palabras[i] == 'b')
{
reverseTxt += "22";
}
else if (palabras[i] == 'c')
{
reverseTxt += "222";
}
else if (palabras[i] == 'd')
{
reverseTxt += "3";
}
else if (palabras[i] == 'e')
{
reverseTxt += "33";
}
else if (palabras[i] == 'f')
{
reverseTxt += "333";
}
else if (palabras[i] == 'g')
{
reverseTxt += "4";
}
else if (palabras[i] == 'h')
{
reverseTxt += "44";
}
else if (palabras[i] == 'i')
{
reverseTxt += "444";
}
else if (palabras[i] == 'j')
{
reverseTxt += "5";
}
else if (palabras[i] == 'k')
{
reverseTxt += "55";
}
else if (palabras[i] == 'l')
{
reverseTxt += "555";
}
else if (palabras[i] == 'm')
{
reverseTxt += "6";
}
else if (palabras[i] == 'n')
{
reverseTxt += "66";
}
else if (palabras[i] == 'o')
{
reverseTxt += "666";
}
else if (palabras[i] == 'p')
{
reverseTxt += "7";
}
else if (palabras[i] == 'q')
{
reverseTxt += "77";
}
else if (palabras[i] == 'r')
{
reverseTxt += "777";
}
else if (palabras[i] == 's')
{
reverseTxt += "7777";
}
else if (palabras[i] == 't')
{
reverseTxt += "8";
}
else if (palabras[i] == 'u')
{
reverseTxt += "88";
}
else if (palabras[i] == 'v')
{
reverseTxt += "888";
}
else if (palabras[i] == 'w')
{
reverseTxt += "9";
}
else if (palabras[i] == 'x')
{
reverseTxt += "99";
}
else if (palabras[i] == 'y')
{
reverseTxt += "999";
}
else if (palabras[i] == 'z')
{
reverseTxt += "9999";
}
else if (palabras[i] == ' ')
{
reverseTxt += "0";
}
}
this.textBox2.Text = reverseTxt;
}
}
}
3 - C++
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int n1, n2, n3;
int maximo;
int i;
n1 = Convert.ToInt32(textBox1.Text);
n2 = Convert.ToInt32(textBox2.Text);
n3 = Convert.ToInt32(textBox3.Text);
maximo = n1;//
if (n2 > maximo)
{
maximo = n2;//
}
if (n3 > maximo)
{
maximo = n3;//
}
i = maximo;//
while ((i % n1 != 0) || (i % n2 != 0) || (i % n3 != 0))
{
i++;
}
textBox4.Text = i.ToString();
}
}
}
4 - F++
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio4
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int[] primos = new int[1000000]; int cliente;
cliente = Convert.ToInt32(textBox1.Text);
for (int numero = 0; numero < 1000000; numero++)
{
string pal1 = Convert.ToString(numero);
string pal2 = "";
string le = "";
int tl = 0;
int k = 0;
tl = pal1.Length;
for (k = tl - 1; k >= 0; k--)
{
le = pal1.Substring(k, 1);
pal2 = pal2 + le;
}
int div = 0;
for (int i = 1; i <= 9; i++)
{
if (numero % i == 0)
div++;
}
if (div == 1 && pal1.Equals(pal2) || (numero < 10 && div == 2) && pal1.Equals(pal2))
{
primos[numero] = numero;
}
}
// MessageBox.Show("EL ARRAY SE A LLENADO");
for (int h = 0; h < primos.Length ; h++)
{
if (primos[h] != 0 && primos[h] > cliente)
{
textBox2.Text = primos[h].ToString();
break;
}
else
{
}
}
}
}
}
5 - PASCAL
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejericio5
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
menu();
}
public static int Segmentos_Encendidos(string hora)
{
int encendidos = 0;
for (int f = 0; f < 6; f++)
{
encendidos += segmentos[(int)char.GetNumericValue(hora[f])];
}
return encendidos;
}
public static string Convertir_cadena(int numero)
{
string retorno;
retorno = Convert.ToString(numero);
if (retorno.Length == 1)
{
retorno = "0" + retorno;
}
return retorno;
}
public static int[] segmentos = new int[] { 6, 2, 5, 5, 4, 5, 6, 3, 7, 6 };
public void menu()
{
int hora = 0, minutos = 0, segundos = 0;
int segundos_transcurridos;
int leds_encendidos = 0;
string hora_actual;
segundos_transcurridos = Convert.ToInt32(textBox1.Text);
while (segundos_transcurridos > -1)
{
hora_actual = Convertir_cadena(hora) + Convertir_cadena(minutos) + Convertir_cadena(segundos);
leds_encendidos += Segmentos_Encendidos(hora_actual);
segundos++;
if (segundos == 60)
{
segundos = 0;
minutos++;
if (minutos == 60)
{
minutos = 0;
hora++;
if (hora > 23)
{
hora = 0;
}
}
}
segundos_transcurridos--;
}
textBox2.Text = Convert.ToString(leds_encendidos);
}
}
}
6 - COBOL
ES EL MISMO QUE EL 1
7 - ARDUINO
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio7
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string linea;
linea = textBox1.Text;
linea = linea.Trim();
while (linea.IndexOf(" ") >= 0)
{
linea.Replace(" ", " ");
}
string[] numeros = linea.Split(new char[] { ' ' });
long suma = 0;
foreach (string numero in numeros)
suma += Convert.ToInt64(numero);
textBox2.Text = suma.ToString();
}
}
}
9 - MICRO C
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio9
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int segundos, minutos, horas, dias;
segundos = Convert.ToInt32(textBox1.Text);
minutos = segundos / 60;
horas = segundos / 3600;
dias = segundos / 86400;
textBox2.Text = minutos.ToString();
textBox3.Text = horas.ToString();
textBox4.Text = dias.ToString();
}
}
}
DIFICILESSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS
1* - C++
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Random rnd = new Random();
int jugador1 = rnd.Next(1,3);
int jugador2 = rnd.Next(1, 3);
int jugador3 = rnd.Next(1, 3);
//JUGADOR1
if (jugador1 == 1)
{
textBox1.Text = "Escudo";
}
else if (jugador1 == 2)
{
textBox1.Text = "Corona";
}
//JUGADOR2
if (jugador2 == 1)
{
textBox2.Text = "Escudo";
}
else if (jugador2 == 2)
{
textBox2.Text = "Corona";
}
//JUGADOR3
if (jugador3 == 1)
{
textBox3.Text = "Escudo";
}
else if (jugador3 == 2)
{
textBox3.Text = "Corona";
}
if (jugador1 == 1 && jugador2 == 1 && jugador3 == 1 || jugador1 == 2 && jugador2 == 2 && jugador3 == 2)
{
textBox4.Text = "Empate nadie gana";
}
if (jugador1 > jugador2 && jugador1 > jugador3 || jugador1 < jugador2 && jugador1 < jugador3)
{
textBox4.Text = "JUGADOR 1 GANA";
}
if (jugador2 > jugador1 && jugador2 > jugador3 || jugador2 < jugador1 && jugador2 < jugador3)
{
textBox4.Text = "JUGADOR 2 GANA";
}
if (jugador3 > jugador2 && jugador3 > jugador1 || jugador3 < jugador2 && jugador3 < jugador1)
{
textBox4.Text = "JUGADOR 3 GANA";
}
}
}
}
2* - JAVA
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string cadena1, cadena2;
cadena1 = textBox1.Text;
cadena2 = textBox2.Text;
int L;
int tam1, tam2,pos1=0,pos2=0;
tam1 = cadena1.Length;
tam2 = cadena2.Length;
if (cadena1.Length > cadena2.Length)
{
L = cadena1.Length;
}
else
{
L = cadena2.Length;
}
string letra = "";
for (int y = 0; y < L; y=y+2)
{
if (y < cadena1.Length)
{
if (tam1 > 1)
{
letra = letra + cadena1.Substring(pos1, 2);
tam1 = tam1 - 2;
pos1 = pos1 + 2;
}
else
{
letra = letra + cadena1.Substring(pos1, 1);
pos1 = pos1 + 2;
}
}
if (y < cadena2.Length)
{
if (tam2 > 1)
{
letra = letra + cadena2.Substring(pos2, 2);
tam2 = tam2 - 2;
pos2 = pos2 + 2;
}
else
{
letra = letra + cadena2.Substring(pos2, 1);
pos2 = pos2 + 2;
}
}
}
textBox3.Text = letra;
}
}
}
3* - TURBOPASCAL
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string texto = textBox1.Text;
string pigLatin = "";
string primerLetra;
string restoDeLaPalabra;
string vocales = "AEIOUaeiou";
int PosLetra;
foreach (string word in texto.Split())
{
int mol = 0;
char[] words = texto.ToCharArray();
for (int i = 0; i < words.Length; i++)
{
if (words[i] == 'a' || words[i] == 'e' || words[i] == 'i' || words[i] == 'o' || words[i] == 'u')
{
break;
}
else
{
mol++;
}
}
primerLetra = word.Substring(0, mol);
restoDeLaPalabra = word.Substring(primerLetra.Length);
PosLetra = vocales.IndexOf(primerLetra);
if (PosLetra == -1)
{
pigLatin = restoDeLaPalabra + primerLetra + "ay";
}
else
{
pigLatin = word + "way";
}
textBox2.Text = pigLatin;
textBox1.Focus();
}
}
}
}
4* - F++
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio4
{
public partial class Form1 : Form
{
int intentos, comparacion;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int usuario;
usuario = Convert.ToInt32(textBox1.Text);
textBox2.Text = comparacion.ToString();
if (usuario == comparacion)
{
textBox3.Text = "FELICIDADES ACERTO EL NUMERO";
intentos++;
}
else if (usuario > comparacion)
{
textBox3.Text = "TE PASASTE";
intentos++;
}
else if (usuario < comparacion)
{
textBox3.Text = "MUY BAJO";
intentos++;
}
textBox4.Text = intentos.ToString();
}
private void button2_Click(object sender, EventArgs e)
{
Random rdm = new Random();
comparacion = rdm.Next(0, 101);
MessageBox.Show("EL NUMERO A SIDO GENERADO");
}
}
}
5 - FINAL
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Ejercicio5
{
public partial class Form1 : Form
{
double nuevonum = 0;
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
textBox2.Text = "";
double numfinal = 0;
numfinal = Convert.ToDouble(textBox1.Text);
bool esfeliz = false;
for (int x = 0; x < 20; x++)
{
if (Convert.ToInt32(numfinal) == 1)
{
textBox2.Text = "Número Feliz";
esfeliz = true;
}
else
{
numfinal = elevaNum(numfinal);
}
}
if (esfeliz == false)
{
textBox2.Text = "No se pudo calcular el número feliz";
}
}
public double elevaNum(double num)
{
double numele;
double numb;
double nu=0;
string val = num.ToString();
int tam = val.Length;
for (int y = 0; y < tam; y++)
{
numb = Convert.ToDouble(val.Substring(y, 1));
numele = Math.Pow(numb, 2);
nu = nu + numele;
nuevonum = nu;
}
return nuevonum;
}
}
}